프로그래밍 시작하기: 파이썬 입문(Inflearn Original) Project
2023. 1. 15. 23:53ㆍPython
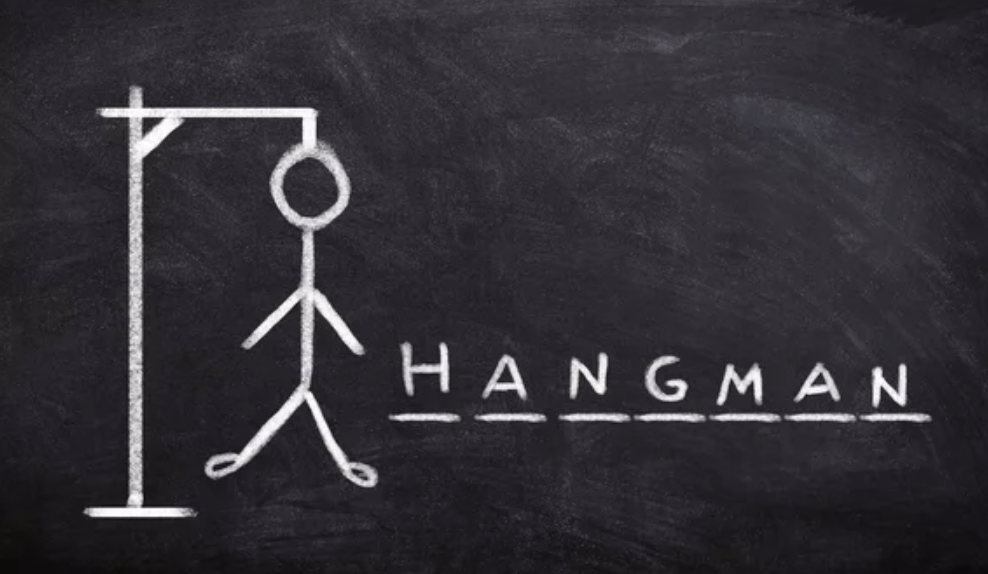
Hangman(행맨) 미니 게임 제작(1)
# 기본 프로그램 제작 및 테스트
import time
# 처음 인사
name = input("What is your name?")
print("Hi, " + name, "Time to play hangman game!")
print()
time. sleep(1)
print("Start Loading...")
print()
time.sleep(0.5)
# 정답 단어
word = "secret"
# 추측 단어
guesses = ''
# 기회
turns = 10
# 핵심 While Loop
# 찬스 카운트가 남아 있을 경우
while turns > 0:
# 실패 횟수
failed = 0
# 정답 단어 반복
for char in word:
# 정답 단어 내에 추측 문자가 포함되어 있는 경우
if char in guesses:
# 추측 단어 출력
print(char, end=' ')
else:
# 틀린 경우 대시로 처리
print("_", end=' ')
failed += 1
#단어 추측이 성공 한 경우
if failed == 0:
print()
print()
print('Congratulations! The Guesses is correct.')
# while 구문 중단
break
print()
# 추측 단어 문자 단위 입력
print()
guess = input("guess a character")
# 단어 더하기
guesses += guess
# 정답 단어에 추측한 문자가 포함되어 있지 않으면
if guess not in word:
# 기회 회수 감소
turns -= 1
# 오류 메시지
print("Oops! Wrong")
# 남은 기회 출력
print("You have", turns, 'more guesses!')
if turns == 0:
# 실패 메시지
print("You hangman game failed. Bye!")
What is your name?kim
Hi, kim Time to play hangman game!
Start Loading...
_ _ _ _ _ _
guess a charactere
_ e _ _ e _
guess a characterc
_ e c _ e _
guess a characterr
_ e c r e _
guess a characters
s e c r e _
guess a charactert
s e c r e t
Congratulations! The Guesses is correct.
Hangman(행맨) 미니 게임 제작(2)
# 프로그램 완성 및 최종 테스트
# 실습 사운드 모듈(mac) 수정
import time
# csv 처리
import csv
# 랜덤
import random
# 사운드 처리
!pip install pygame
import pygame
# 처음 인사
name = input("What is your name?")
print("Hi, " + name, "Time to play hangman game!")
print()
time. sleep(1)
print("Start Loading...")
print()
time.sleep(0.5)
# CSV 단어 리스트
words = []
# 문제 CSV 파일 로드
with open('/content/word_list.csv', 'r') as f:
reader = csv.reader(f)
# Header Skip
next(reader)
for c in reader:
words.append(c)
# 리스트 섞기
random.shuffle(words)
#임의의 단어 선택
q = random.choice(words)
# 정답 단어
word = q[0].strip()
# 추측 단어
guesses = ''
# 기회
turns = 10
# 핵심 While Loop
# 찬스 카운트가 남아 있을 경우
while turns > 0:
# 실패 횟수
failed = 0
# 정답 단어 반복
for char in word:
# 정답 단어 내에 추측 문자가 포함되어 있는 경우
if char in guesses:
# 추측 단어 출력
print(char, end=' ')
else:
# 틀린 경우 대시로 처리
print("_", end=' ')
failed += 1
#단어 추측이 성공 한 경우
if failed == 0:
print()
print()
print('Congratulations! The Guesses is correct.')
# while 구문 중단
break
print()
# 추측 단어 문자 단위 입력
print()
print('Hint : {}'.format(q[1].strip()))
guess = input("guess a character")
# 단어 더하기
guesses += guess
# 정답 단어에 추측한 문자가 포함되어 있지 않으면
if guess not in word:
# 기회 회수 감소
turns -= 1
# 오류 메시지
print("Oops! Wrong")
# 남은 기회 출력
print("You have", turns, 'more guesses!')
if turns == 0:
# 실패 메시지
print("You hangman game failed. Bye!")
Looking in indexes: https://pypi.org/simple, https://us-python.pkg.dev/colab-wheels/public/simple/
Requirement already satisfied: pygame in /usr/local/lib/python3.8/dist-packages (2.1.2)
What is your name?kim
Hi, kim Time to play hangman game!
Start Loading...
_ _ _ _ _ _
Hint : fruit
guess a characterc
Oops! Wrong
You have 9 more guesses!
_ _ _ _ _ _
Hint : fruit
guess a charactern
_ _ _ n _ _
Hint : fruit
guess a charactere
_ _ _ n _ e
Hint : fruit
guess a characterr
_ r _ n _ e
Hint : fruit
guess a characters
Oops! Wrong
You have 8 more guesses!
_ r _ n _ e
Hint : fruit
guess a charactera
_ r a n _ e
Hint : fruit
guess a charactero
o r a n _ e
Hint : fruit
guess a characterg
o r a n g e
Congratulations! The Guesses is correct.